Controls
Controls are defined using JSON Schema or Zod, providing a strong run-time validation system for your workflows.
This ensures that you as the developer and your non-technical peers are speaking the same language. Those responsible for styling and copy can edit with confidence, knowing their changes are tested in code.
Controls vs Payload
Control Schema - For Non-Technical Peers and Developers. Managed in the Novu Dashboard UI, defined by developers and used by non-technical peers.
Payload Schema - For Developers. Passed during the novu.trigger
method, and controlled by the developer.
Common usecases
- Content - Modify any static content: email subject, email body, push notification title, etc…
- Styling - Modify the styling of the content: button color, background color, font size, etc…
- Behaviour - Modify the behaviour of the content: show/hide a section, show/hide a button, etc…
- Order - Modify the order of the content: the order of the email sections, the order of the buttons, etc…
- Actions - Modify the behaviour of actions: digest duration, etc…
- Other - Any other use case that should be controller without modifying code
Step Controls
Step Control schema defines the control passed during the step
method. These controls can be modified and persisted in the Novu Dashboard UI.
The snippet below shows a configuration for the Step Control schema. If you don’t provide a schema, Typescript will infer the data type to unknown
, reminding you of the best practice to specify your schema.
For the full list of parameters, check out the full SDK reference.
Supported Schema Types
- Zod Schema - A TypeScript-first schema declaration and validation library.
- JSON Schema - The most popular schema language for defining JSON data structures.
Using Variables
To facilitate the use of variables in the control schema, enclose the variable name in double curly braces using the {{variableName}}
syntax. For example, {{subscriber.firstName | capitalize}}
will be dynamically replaced with the actual value of the subscriber’s first name at runtime.
You can use variables in any step control value, whether set by the developer or within the Novu Dashboard UI. To facilitate this, the Novu Dashboard UI offers auto-completion for variables. Simply start typing {{
to view a list of all available variables.
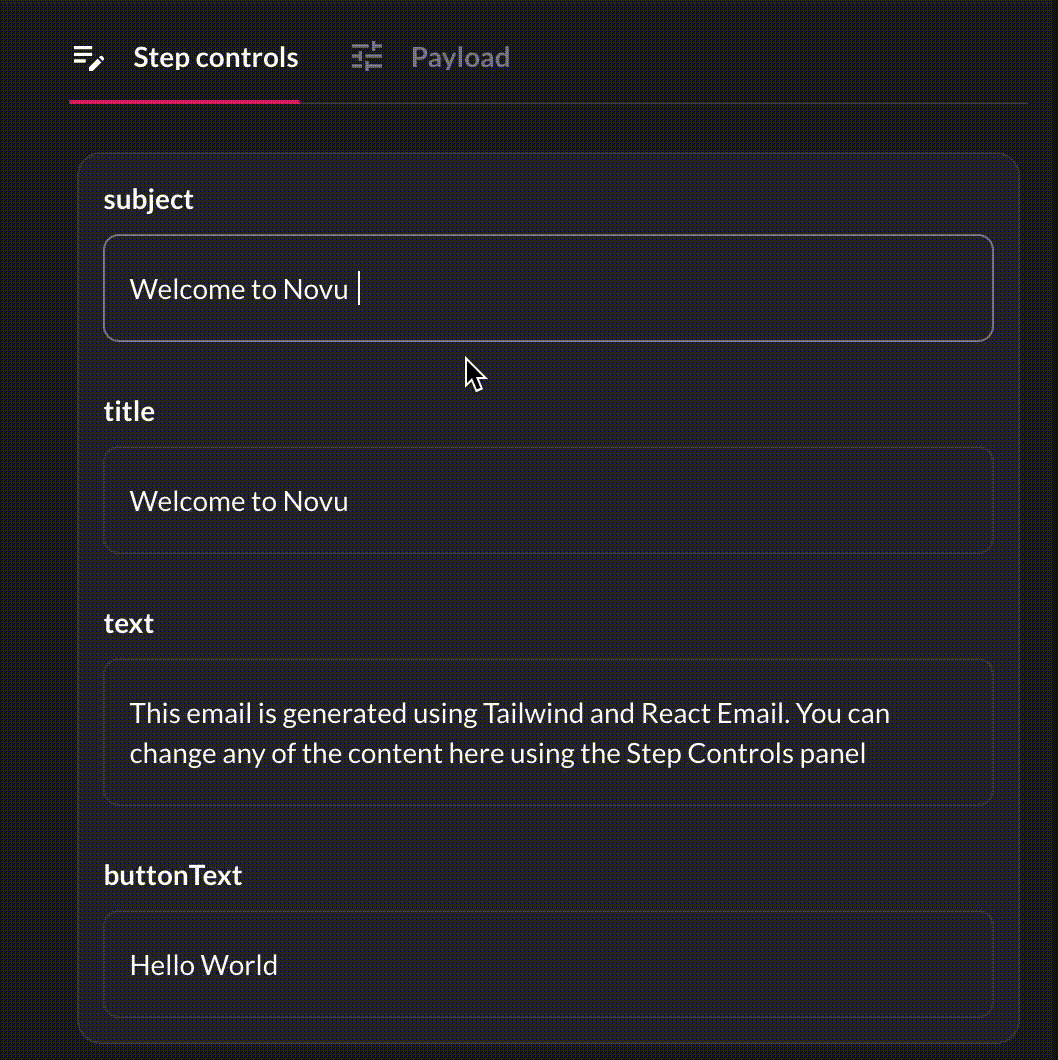
Example for variables autocomplete in dashboard
Variable Options
- Subscriber Attributes: Access all subscriber attributes. Example:
{{subscriber.firstName}}
- Payload Variables: Use all payload variables defined in the
payloadSchema
. Example:{{payload.userId}}
- Liquid Filters: Apply liquid filters to format or manipulate variable values. Examples:
{{subscriber.firstName | append: ': ' | append: payload.status | capitalize}}
or{{payload.invoiceDate | date: '%a, %b %d, %y'}}
will format the date asThu, Jan 01, 24