Styling the Inbox component
Learn how to style the pre built Inbox component
Customization Hierarchy
The Inbox component is built to allow for multiple layers of styling, which allows the specificity required to style the Inbox to meet the requirements of your use case.
Depending on the level of customization you need, you can choose to style the inbox using one of the following approaches:
- Appearance Prop
- Custom notification rendering - Render a custom notification item with complete control
- Custom Composition - Compose our components for custom layouts
You can see Styling in action on the Inbox Playground with common themes like Notion, Reddit and more!
Appearance Prop
The appearance
prop can be used to customise the Inbox. It has three main keys: baseTheme
, variables
and elements
.
- Variables: Global styling variables that apply to multiple elements within the inbox.
- Elements: Elements are the individual UI components that make up the Inbox.
- Base theme: This is the base theme applied to the
<Inbox />
. It has the same keys asappearance
. Used for applying base themes likedark
.
Variables
Variables are used to define global styling properties that can be reused throughout the inbox.
You might want to use variables to the styling of multiple components at once, for example, if you want to change the border radius of all the components at once, you can do so by updating the colorPrimary
variable, which will modify the CTA buttons, unseen counter and etc...
Prop | Type | Default |
---|---|---|
colorBackground? | string | - |
colorForeground? | string | - |
colorPrimary? | string | - |
colorPrimaryForeground? | string | - |
colorSecondary? | string | - |
colorSecondaryForeground? | string | - |
colorCounter? | string | - |
colorCounterForeground? | string | - |
colorNeutral? | string | - |
colorShadow? | string | - |
fontSize? | string | - |
borderRadius? | string | - |
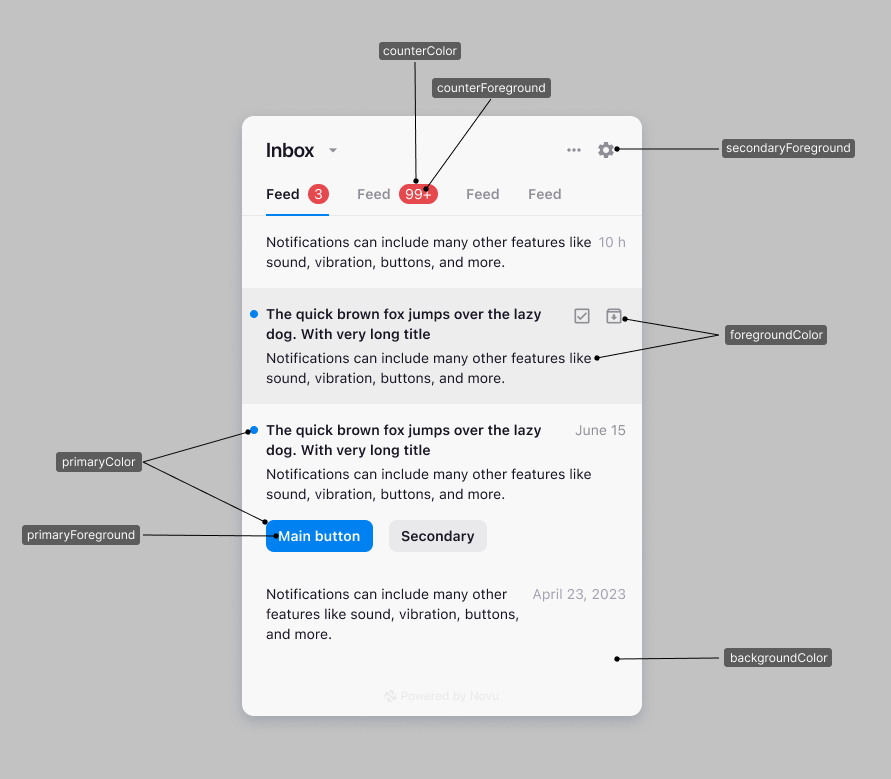
Styling Variables
You can override the default elements by passing your own styles or CSS classes to the elements
object.
Elements
The elements
object allows you to define styles for these components. Each key corresponds to an component, and the value is an object containing style properties
or you can also pass your css classes
.
Here's a list of available elements that can be styled using the elements
object in your appearance configuration:
Finding element selectors
You can inspect the elements you want to customize using the browser's dev tools, each element has a unique selector that you can use to style starting with nv-
.
Strip the nv-
prefix when and add it to the elements
object. For example, to style the nv-notificationPrimaryAction__button
element, you can add the following to the elements
object:
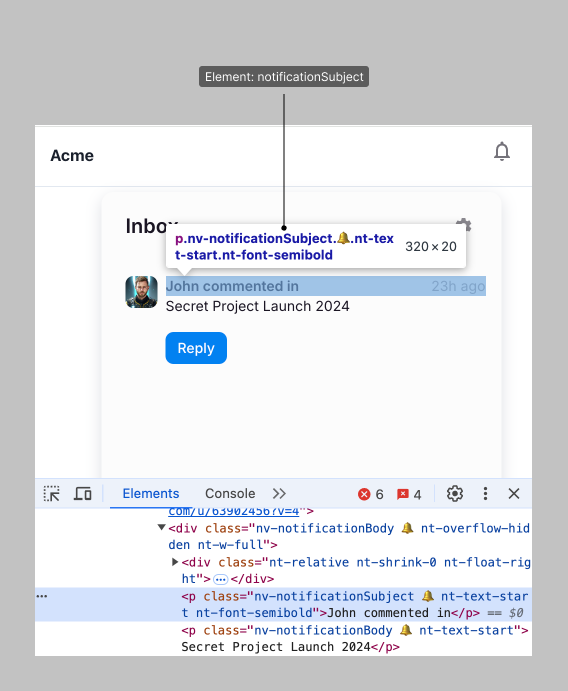
Any selector that appears before the 🔔 emoji, can be targeted via the elements property in
Appearance prop (stripping the nv-
prefix). You can also use TS autocomplete to find the
available elements.
Dark theme
No need to implement a custom dark theme. Just import our premade dark
theme and use it via the baseTheme
option in appearance
.
Bring your own CSS
You can override the default elements by passing your own styles or CSS classes to the elements
object.
Using Tailwind CSS
You can also use Tailwind CSS classes to style the Inbox components. You can pass the classes
directly to the elements
object.
Using CSS Modules
You can also use CSS Modules
to style the Inbox components. Here's how you can do it:
- Create a CSS module file
(e.g. styles.module.css)
with the styles you want to apply to the Inbox components.
- Import the CSS module file and pass the classes to the elements object.
Using Styles Object
You can also use a styles object to style the Inbox components. You can pass the styles
directly to the elements
object.
Render subject and body with bold text
By default, the Inbox notification text for the subject and body is rendered in a normal font weight. To highlight important words or phrases within your notification messages, you can wrap the desired subject or body text in double asterisks (**). Here's an example of how you can do this using the Novu Framework:
Please note, that when you are rendering the custom notification component you will need to parse the text and apply the bold styling accordingly.
Currently we only support bold text. We are working on adding more text formatting options in the future. You can learn more about the Novu Framework in-app step here.
Render Notification Component
You can render your own custom notification item component by passing a renderNotification
prop to the Inbox
or Notifications
component, this will allow you to style and render more complex notification items.