React Preferences Component
Learn how to use and customize the Preferences component in React to manage notification settings.
By default, Novu will show the subscriber preferences cog icon on the Inbox component.
Users can enable/disable any active channel in the workflow using subscriber preferences or they can update the preference globally for all workflows under the Global Preferences
.
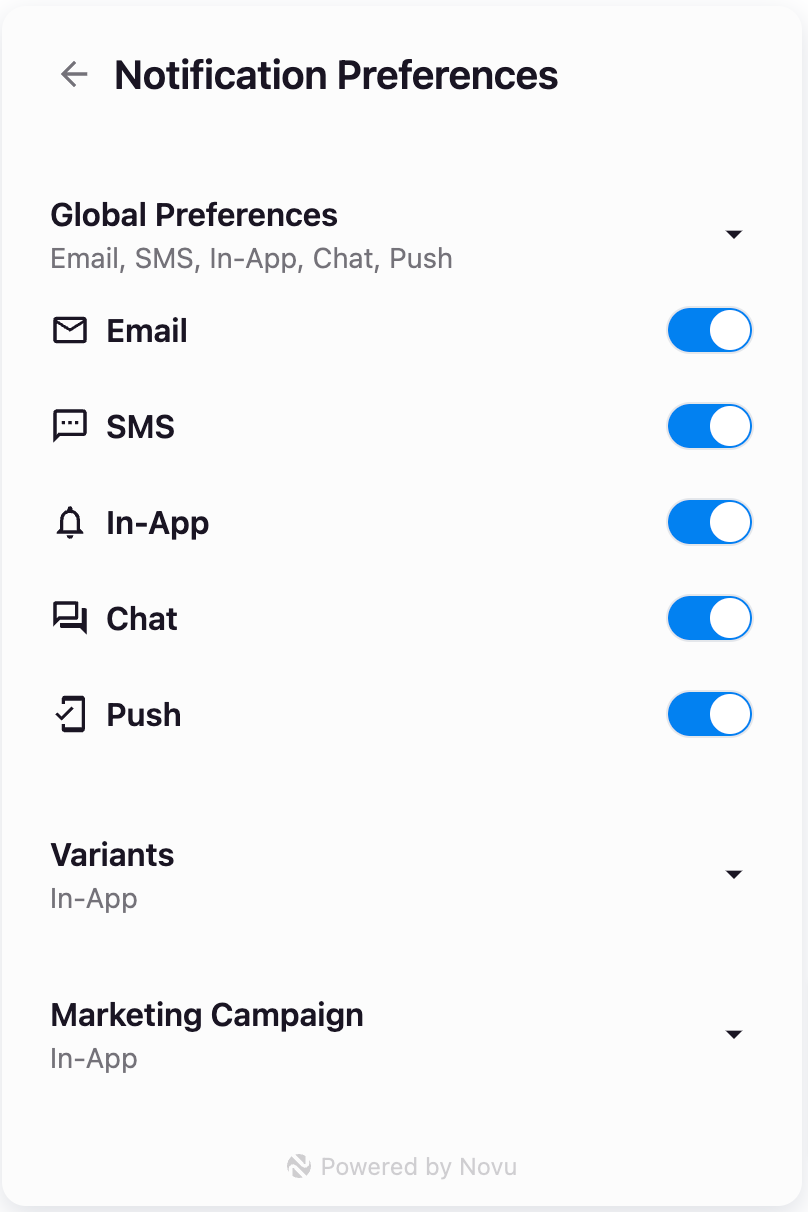
Basic Usage
The Preferences
component is used to display the subscriber preferences. Use it when you want to render preferences in another part of your application or create a custom layout for the Inbox.
Customization Options
Filtering Preferences
You can filter the preferences visible by the user by specifying the preferenceFilter
on the
Inbox
component. Learn more about it
here.
Hiding Global Preferences
Global subscriber preferences are shown by default. To hide them, add the following code to your global CSS file: